Oracle has provided an excellent document on the same
http://docs.oracle.com/javase/tutorial/uiswing/dnd/intro.html
With this feature in mind lets create a project to move data from one table to another using drag and drop feature.
Lets create two table and insert some record in to one of the table.
create table empTable1 (Name varchar2(20),EmpId varchar2(10),Dept varchar2(20));
create table empTable2 (Name varchar2(20),EmpId varchar2(10),Dept varchar2(20));
insert into empTable1 values('Arpit','1234','Oracle');
insert into empTable1 values('Chandan','1233','EBS');
insert into empTable1 values('Ankit','1232','Andriod');
insert into empTable1 values('Nitin','1231','Robotics');
insert into empTable1 values('Anil','1230','C++');
insert into empTable1 values('Deepak','1229','Toolkit');
insert into empTable1 values('Harendra','1228','Mobile');
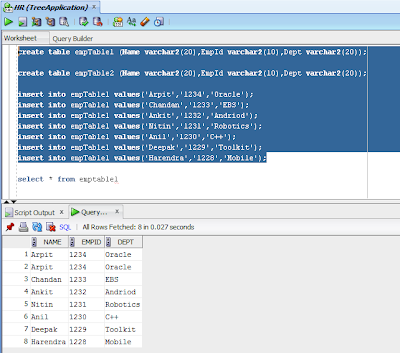
In this exercise we will just try to reorder the rows by just dragging and dropping the rows. So we will use one table for this purpose.
Later we will use the same exercise and extend it to drop the rows in different table.
Create a Business component from table
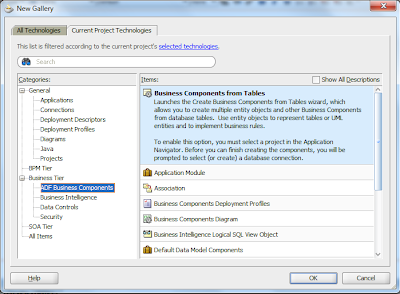
Connect to schema where you have created your tables
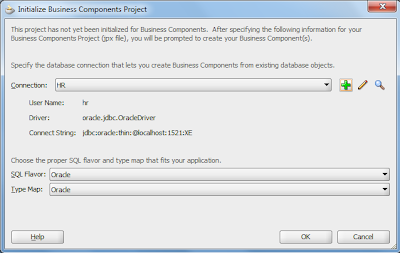
Select your tables
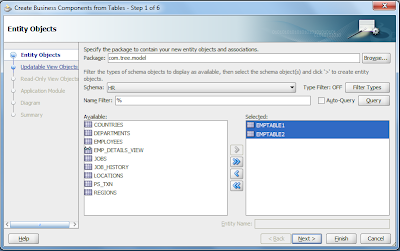
Create EO and VO and finish your wizard.
Now create a jsf page and drag and drop the two table in to the page so that your page should appear like this.
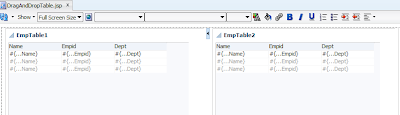
We are adding both the tables however in this exercise we will use only the first table where we have the data.
We will try to drag and drop the rows from the first table itself and while dropping in to the table we will change the column values.
Once the page is created search for a DragSource and drag and drop it into the first table.
ONce it is dropped you can go to property inspector to define the action that has to be perfomred.
Select action as MOVE and specify a meaningful value to Discriminant
The discriminant property allows the user to cor-relate a drag event with corresponding drop target so that in case of multiple drag and drop the user do not get confused.
Finally select the DragDropEndListener and create a Bean and a method for drag source listener
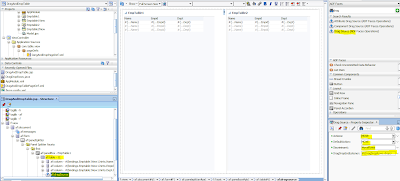
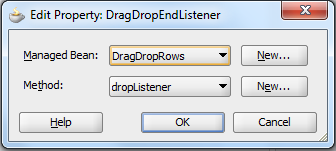
Search for Drop now in the component pallete.
Drag and drop Collection Drop target(as we are dragging rows) and drop it to the same table as shown.
Ensure the model name matches the discriminant that you have specified in the Dragsource
Click on the DropListener and create new method for the dropListener in the same bean you have created earlier.
Save all the changes.
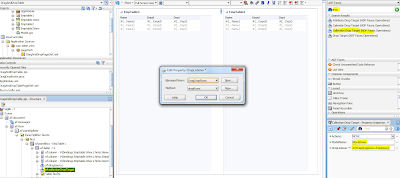
Now everything is java. We want that as soon as the dragged row is dropped the select rows gets deleted from the source node . This can be implemented using the following code
public void dropListener(DropEvent dropEvent) {
// Add event code here...
DCBindingContainer bc =
(DCBindingContainer)BindingContext.getCurrent().getCurrentBindingsEntry();
DCIteratorBinding dcib =
bc.findIteratorBinding("Emptable1View1Iterator");
RowSetIterator iter = dcib.getRowSetIterator();
iter.removeCurrentRow();
}
ReferenceOnce we are able to delete the source row we have selected we can now simultaneously go to the drop target listener.
For drop target listener we have the following code
public DnDAction dropRows(DropEvent dropEvent) {
// Add event code here...
RichTable rt = (RichTable)dropEvent.getDragComponent();
Transferable t = dropEvent.getTransferable();
DataFlavor
df =
DataFlavor.getDataFlavor(RowKeySet.class, "MoveRows");
RowKeySet rowKeySet = t.getData(df);
List key = (List)rowKeySet.iterator().next();
rt.setRowKey(key);
JUCtrlHierNodeBinding rowBinding =(JUCtrlHierNodeBinding)rt.getRowData();
Row row = (Row)rowBinding.getRow();
//addRow(row);
DCBindingContainer bc =
(DCBindingContainer)BindingContext.getCurrent().getCurrentBindingsEntry();
DCIteratorBinding dc =
bc.findIteratorBinding("Emptable1View1Iterator");
RowSetIterator iter = dc.getRowSetIterator();
Row newRow = iter.createRow();
newRow.setAttribute("Name", row.getAttribute("Name"));
newRow.setAttribute("Empid", row.getAttribute("Dept"));
newRow.setAttribute("Dept", row.getAttribute("Empid"));
iter.insertRowAtRangeIndex(iter.getRowCount(), newRow);
return DnDAction.MOVE;
}
It get the data from the dragged source and then as you can see it create a new rows and you can notice one thing in the code
newRow.setAttribute("Name", row.getAttribute("Name"));
newRow.setAttribute("Empid", row.getAttribute("Dept"));
newRow.setAttribute("Dept", row.getAttribute("Empid"));
The new node thus created will have Dept value in Empid column and vice versa.
Lets deploy the code and test it from the console.you will get a page like this

Now try dragging and droppin any rows in the table.
I dragged the first field and droppped it in the same table and i got the following result.
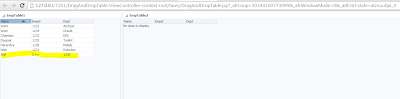
As you can obvserver the row which i have dragged and dropped the value for empid and dept are interchanged. This is what we were trying to achieve in this exercise.The current code is working fine only for one row.
Probably not a great example but it must have given you a basic idea as how drag and drop of table works. We will now extend the same sample and try to achieve different functionalities in the same such as dragging and dropping multiple rows, moving data from one table to another.